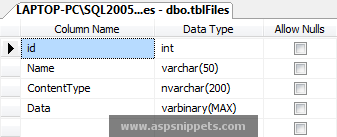
HTML Markup
The HTML Markup contains a FileUpload and Button to upload and save the files to database and an ASP.Net GridView control to display the uploaded files and also to allow the user to download the file saved in database. The ID of the File is bound to the CommandArgument property of the LinkButton. it will later be used to download the file.
<asp:FileUpload ID="FileUpload1" runat="server" />
<asp:Button ID="btnUpload" runat="server" Text="Upload" OnClick="Upload" />
<hr />
<asp:GridView ID="GridView1" runat="server" HeaderStyle-BackColor="#3AC0F2" HeaderStyle-ForeColor="White"
RowStyle-BackColor="#A1DCF2" AlternatingRowStyle-BackColor="White" AlternatingRowStyle-ForeColor="#000"
AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="Name" HeaderText="File Name"/>
<asp:TemplateField ItemStyle-HorizontalAlign = "Center">
<ItemTemplate>
<asp:LinkButton ID="lnkDownload" runat="server" Text="Download" OnClick="DownloadFile"
CommandArgument='<%# Eval("Id") %>'></asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
Namespaces
You will need to import the following namespaces
C#
using System.IO;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
VB.Net
Imports System.IO
Imports System.Data
Imports System.Data.SqlClient
Imports System.Configuration
Uploading the files and then saving in SQL Server Database table
The below event handler gets executed when the Upload Button is clicked, it simply saves the file as Binary data in the SQL Server Database
The name of the file, the content type (MIME type) and the actual file as array of bytes are inserted into the database table.
Note: The Content type (MIME type) is very important while downloading the files as it notifies the browser about type of the File
C#
protected void Upload(object sender, EventArgs e)
{
string filename = Path.GetFileName(FileUpload1.PostedFile.FileName);
string contentType = FileUpload1.PostedFile.ContentType;
using (Stream fs = FileUpload1.PostedFile.InputStream)
{
using (BinaryReader br = new BinaryReader(fs))
{
byte[] bytes = br.ReadBytes((Int32)fs.Length);
string constr = ConfigurationManager.ConnectionStrings["constr"].ConnectionString;
using (SqlConnection con = new SqlConnection(constr))
{
string query = "insert into tblFiles values (@Name, @ContentType, @Data)";
using (SqlCommand cmd = new SqlCommand(query))
{
cmd.Connection = con;
cmd.Parameters.AddWithValue("@Name", filename);
cmd.Parameters.AddWithValue("@ContentType", contentType);
cmd.Parameters.AddWithValue("@Data", bytes);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
}
}
}
}
Response.Redirect(Request.Url.AbsoluteUri);
}
No comments:
Post a Comment