Delete data from Database in ASP.NET using C#
In this example we select the employee name from Dropdown List. All the Textboxes will be fill according the record. Now click on delete button. The data will be deleted successfully.
DeleteDataBase.aspx (Design Page):
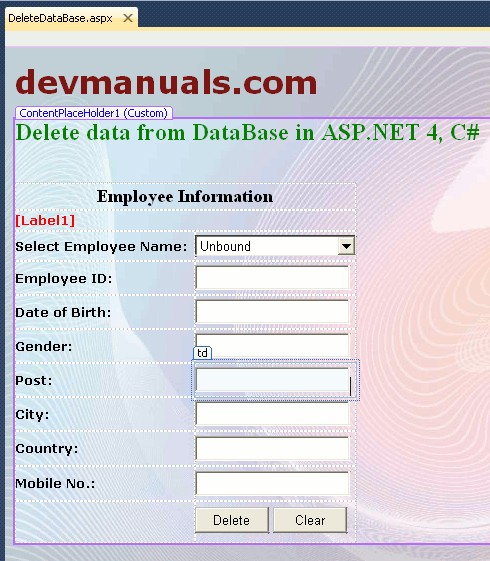
DeleteDataBase.aspx (source code):
View source code Click Here
DeleteDataBase.aspx.cs (C# code file):
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Web.Configuration;
public partial class DeleteDataBase : System.Web.UI.Page
{
SqlConnection con;
SqlCommand cmd;
SqlDataReader dr;
private string s;
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
FillDropDownList();
}
}
// fill dropdownlist
public void FillDropDownList()
{
s = WebConfigurationManager.ConnectionStrings["ChartDatabaseConnectionString"].ConnectionString;
con = new SqlConnection(s);
con.Open();
cmd = new SqlCommand("Select employee_name from Employee", con);
dr = cmd.ExecuteReader();
while (dr.Read())
{
DropDownList1.Items.Add(dr[0].ToString());
}
DropDownList1.Items.Insert(0, new ListItem("Select name for delete record"));
dr.Close();
con.Close();
}
// fill textboxes on select data from dropdownlist
protected void DropDownList1_SelectedIndexChanged(object sender, EventArgs e)
{
s = WebConfigurationManager.ConnectionStrings["ChartDatabaseConnectionString"].ConnectionString;
con = new SqlConnection(s);
con.Open();
cmd = new SqlCommand("Select * from Employee where employee_name ='" + DropDownList1.SelectedItem.ToString() + "'", con);
dr = cmd.ExecuteReader();
while (dr.Read())
{
employee_id_Txt.Text = dr["employee_id"].ToString();
dob_Txt.Text = dr["dob"].ToString();
gender_Txt.Text = dr["gender"].ToString();
post_Txt.Text = dr["post"].ToString();
city_Txt.Text = dr["city"].ToString();
country_Txt.Text = dr["country"].ToString();
mobileno_Txt.Text = dr["mobileno"].ToString();
}
dr.Close();
con.Close();
}
// delete record
protected void delete_Button_Click(object sender, EventArgs e)
{
s = WebConfigurationManager.ConnectionStrings["ChartDatabaseConnectionString"].ConnectionString;
con = new SqlConnection(s);
con.Open();
cmd = new SqlCommand();
cmd = new SqlCommand("delete from Employee where employee_name='" + DropDownList1.SelectedItem.ToString() + "'", con);
cmd.ExecuteNonQuery();
con.Close();
Label1.Text = " Record Deleted successfully";
clearData();
}
// clear textboxes
public void clearData()
{
employee_id_Txt.Text = "";
dob_Txt.Text = "";
gender_Txt.Text = "";
post_Txt.Text = "";
city_Txt.Text = "";
country_Txt.Text = "";
mobileno_Txt.Text = "";
DropDownList1.Items.Clear();
FillDropDownList();
}
// clear form
protected void clear_Button_Click(object sender, EventArgs e)
{
employee_id_Txt.Text = "";
dob_Txt.Text = "";
gender_Txt.Text = "";
post_Txt.Text = "";
city_Txt.Text = "";
country_Txt.Text = "";
mobileno_Txt.Text = "";
DropDownList1.SelectedIndex = 0;
Label1.Text = "";
}
}
Output:
Select Employee Name from Dropdown List. All the Textboxes will be filled according to selected name.

When you click delete button the particular data will be deleted successfully.
Download source code
No comments:
Post a Comment