Simple GridView Example with Row Edit and Delete Options
The GridView control in Asp.Net can be used to display large data in a tabular format. This is a topic which we have discussed earlier. Here in this article we will show you how a GridView control can be used to update (edit), delete and insert data in a remote database.
Recommended for you
The all simple “SqlDataSource” control will be used to bind the GridView with a table in the database. We request you to go through the below link for an overview of how data binding is done using SqlDataSource.
GridView and SqlDataSource Control
Start Microsoft Visual Studio and select “File -> New -> Web Site” from the top menu.
Add a new website and in the “Default.aspx” select the “Design” tab situated at the left bottom. In the design mode, select a GridView and SqlDataSource control. Click the above link and follow the procedure to connect the GridView with a database table. The table will be used to do the transactions.
Run the website, you will see the GridView showing details of employees in tabular format. In this example we have selected 4 columns from the “Employee Details” table.
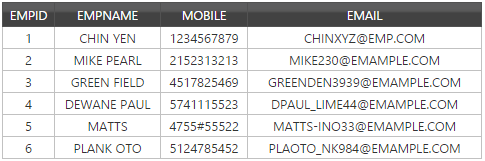
Edit (Update) Row
To edit data in a particular row, add “AutoGenerateEditButton” and set DataKeyNames="EmpID" to the GridView control. The “DataKeyNames” field will be used in the Sql query to edit the employee details.

This will add an “Edit” link button at the beginning of all the rows in the GridView. Clicking the “link” button will do a “PostBack” to the server requesting to edit the row values.
As mentioned earlier, the GridView shows 4 columns. We want to edit selected columns only, say the “Mobile Number” and “Email Address”.
So first set ReadOnly="true" for the fields “EmpID” and “EmpName”.

In “<asp:SqlDataSource />”, add the “UpdateCommand” property, which will have an Sql “Update” command or a procedure to update the selected row’s data. Inside this add 2 parameters (or fields) inside “<UpdateParameters>” property.
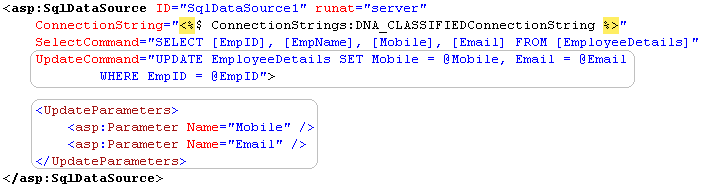
Delete a Row
Similar procedure should be followed to delete one row at a time. Just add AutoGenerateDeleteButton="true" in the GridView control. In the “SqlDataSource” add the below property and parameter.
DeleteCommand="DELETE FROM EmployeeDetails WHERE EmpID = @EmpID"> <DeleteParameters> <asp:Parameter Name="EmpD"/> </DeleteParameters>
That’s it. There is hardly any code to be written to do these transactions. All we need is to write the Sql queries according to our need. But there is one drawback when deleting a particular row. If a user clicks the delete button, the page will post back the delete command and the query is executed which deletes the entire row. (Without any prompt). It can be very disturbing if the row was mistakenly deleted. The lost data cannot come back.
To cease these mistakes from happening, we need a confirmation (yes or no) before deleting any row in the GridView. To accomplish this, set AutoGenerateDeleteButton="false" or remove it entirely.
Delete a Row with a Confirmation
In the “Column” section of the GridView we will add <asp:LinkButton /> control, which will have a client script (Confirm). It will show a popup confirmation message and allows the user to either go with the delete command or negate it.
<Columns>
<asp:TemplateField>
<ItemTemplate>
<asp:LinkButton Runat="server" OnClientClick="return confirm('Are you sure you?');"
CommandName="Delete">Delete</asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
</Columns>
Default.aspx
<%@ Page Language="VB" AutoEventWireup="false" CodeFile="Default.aspx.vb" Inherits="_Default" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title>GridView Edit and Delete Row</title> </head> <body> <form id="form1" runat="server"> <div> <asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" AutoGenerateEditButton="True" AutoGenerateDeleteButton="false" DataSourceID="SqlDataSource1" Font-Names="Arial" Font-Size="Smaller" DataKeyNames="EmpID"> <Columns> <asp:TemplateField> <ItemTemplate> <asp:LinkButton Runat="server" OnClientClick="return confirm('Are you sure you?');" CommandName="Delete" >Delete</asp:LinkButton> </ItemTemplate> </asp:TemplateField> <asp:BoundField DataField="EmpID" HeaderText="EmpID" SortExpression="EmpID" ReadOnly="true" /> <asp:BoundField DataField="EmpName" HeaderText="EmpName" SortExpression="EmpName" ReadOnly="true" /> <asp:BoundField DataField="Mobile" HeaderText="Mobile" SortExpression="Mobile" /> <asp:BoundField DataField="Email" HeaderText="Email" SortExpression="Email" /> </Columns> </asp:GridView> <asp:SqlDataSource ID="SqlDataSource1" runat="server" ConnectionString="<%$ ConnectionStrings:DNA_CLASSIFIEDConnectionString %>" SelectCommand="SELECT [EmpID], [EmpName], [Mobile], [Email] FROM [EmployeeDetails]" UpdateCommand="UPDATE EmployeeDetails SET Mobile = @Mobile, Email = @Email WHERE EmpID = @EmpID" DeleteCommand="DELETE FROM EmployeeDetails WHERE EmpID = @EmpID"> <UpdateParameters> <asp:Parameter Name="Mobile" /> <asp:Parameter Name="Email" /> </UpdateParameters> <DeleteParameters> <asp:Parameter Name="EmpD" /> </DeleteParameters> </asp:SqlDataSource> </div> </form> </body> </html>
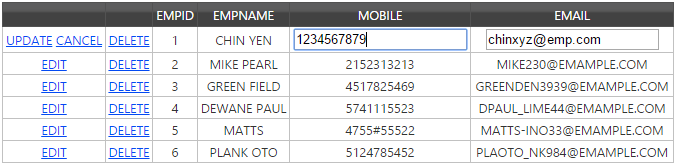
Before running this site on your local host, check if the “web.config” file has been configured properly. To check the file go to “Solution Explorer” and choose the “web.config” file. Inside the file, find <connectionStrings> tag and check if the connection string is properly set. (UserID, Password etc.)
<connectionStrings> <add name="DNA_ConnectionString" connectionString="Data Source=dna; Initial Catalog=DNA_CLASSIFIED;Integrated Security=True" providerName="System.Data.SqlClient"/> </connectionStrings>
No comments:
Post a Comment